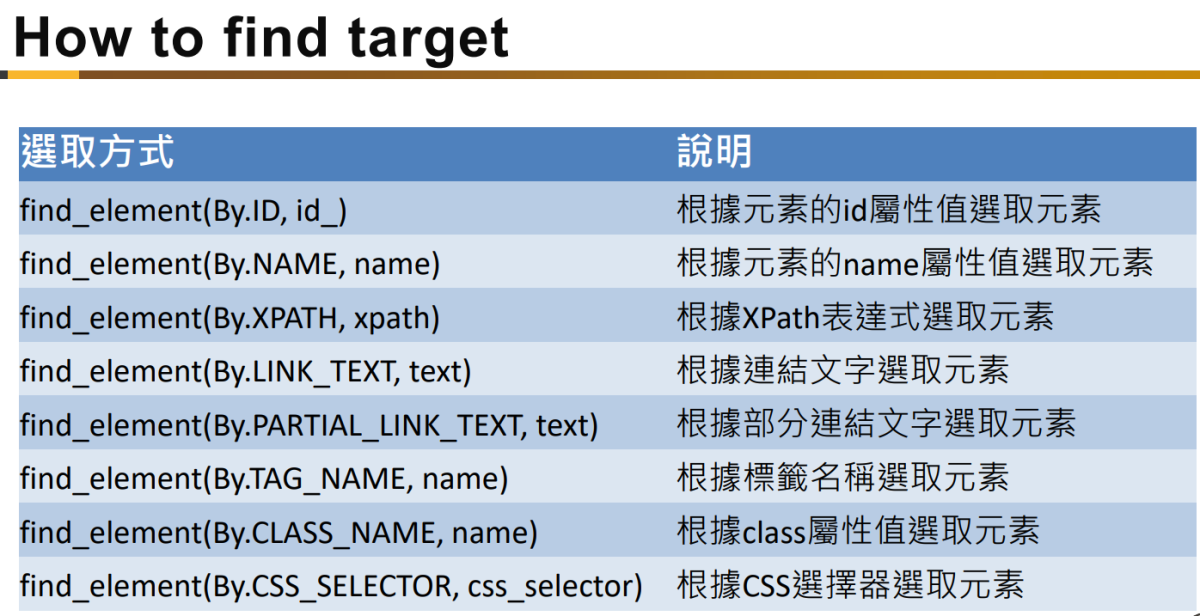
為什麼 標籤名[屬性名=屬性值] 這種格式稱為 CSS_SELECTOR?
這種格式源自於 CSS(Cascading Style Sheets)選擇器 的語法規則。CSS 選擇器被設計用來靈活地選取 HTML 文件中的元素,以便應用樣式。Selenium 借用了這種語法來選取元素,因此這種格式也稱為 CSS_SELECTOR。
CSS 選擇器的基本語法
標籤選擇器(Tag Selector): "div"
選取所有<div>
元素。
屬性選擇器(Attribute Selector): input[type=”text”] 選取所有標籤,且其 type 屬性為 text 的元素。
類選擇器(Class Selector): .class-name 選取所有擁有指定 class 的元素。
ID 選擇器(ID Selector): #id-name 選取擁有指定 id 的元素。
CSS 選擇器能靈活組合這些規則,讓我們能更精確地選取目標元素。
因為 CSS_SELECTOR 是非常靈活且功能強大的選取方式,它可以用來模擬大多數其他選取方式:
1. 模擬 By.TAG_NAME
By.TAG_NAME
:
element = driver.find_element(By.TAG_NAME, "button")
等效的 CSS_SELECTOR
:
element = driver.find_element(By.CSS_SELECTOR, "button")
- 模擬 By.ID
By.ID:
element = driver.find_element(By.ID, "username")
等效的 CSS_SELECTOR
:
element = driver.find_element(By.CSS_SELECTOR, "#username")
3. 模擬 By.CLASS_NAME
By.CLASS_NAME
:
element = driver.find_element(By.CLASS_NAME, "form-control")
等效的 CSS_SELECTOR
:
element = driver.find_element(By.CSS_SELECTOR, ".form-control")
4. 模擬 By.NAME
By.NAME
:
element = driver.find_element(By.NAME, "email")
等效的 CSS_SELECTOR
:
element = driver.find_element(By.CSS_SELECTOR, "input[name='email']")
CSS_SELECTOR 可以完全取代
By.TAG_NAME、By.ID、By.NAME 和 By.CLASS_NAME 的功能,
並且更加靈活。
但是,對於簡單的條件(如 ID 或 Class Name 唯一時),
直接使用專門的方法(如 By.ID)會更直觀和簡潔。
在需要處理複雜結構(如父子層級)時,XPath 仍是不可或缺的工具。
因此,在選取元素時,
可以根據需求靈活使用 CSS_SELECTOR 或其他專門的選取方式。
demo.html內容如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Element Selection Demo</title>
</head>
<body>
<h1 id="main-title" class="header">Welcome to the Demo</h1>
<input type="text" id="username" name="username" class="input-field" placeholder="Enter your username">
<input type="password" id="password" name="password" class="input-field" placeholder="Enter your password">
<button class="btn submit-btn" id="submit-btn">Submit</button>
<a href="https://example.com" class="link" id="example-link">Visit Example</a>
</body>
</html>
使用瀏覽器開啟:
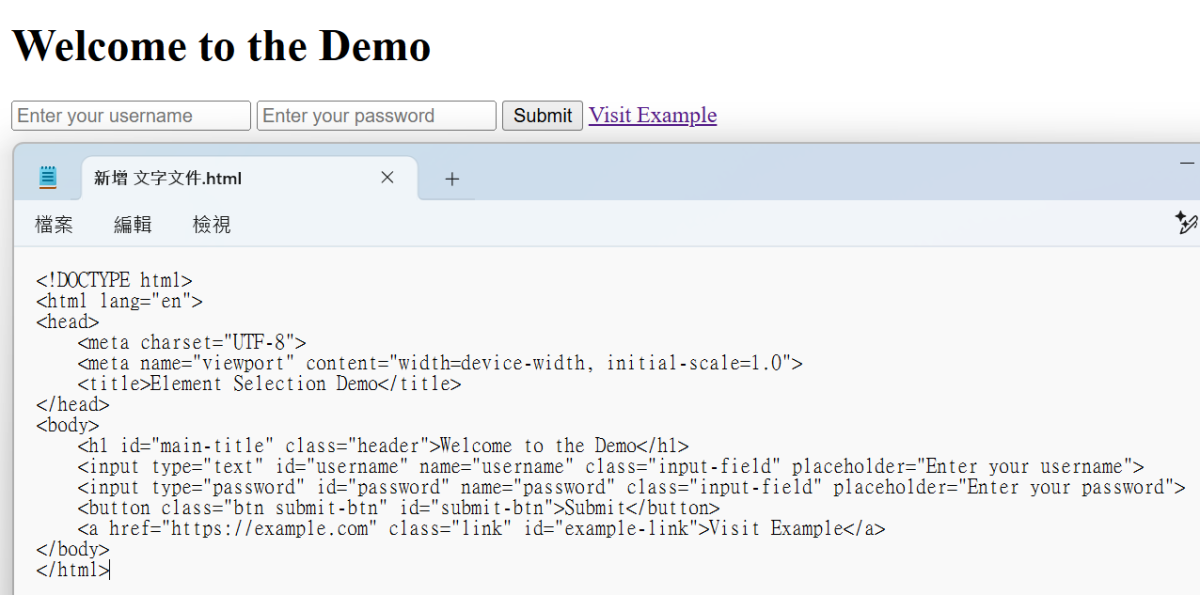
- 使用 Selenium 原生方法
以下使用 By.TAG_NAME、By.ID 等方法選取元素。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.chrome.service import Service
import os
dirname = r"D:\Temp"
basename = "demo.html"
path= os.path.join(dirname,basename)
#'D:\\Temp\\新增 文字文件.html'
driver_path = r"D:\GoogleChromePortable\131.0.6778.264\chromedriver-win64\chromedriver.exe"
services = Service(executable_path= driver_path )
#<selenium.webdriver.chrome.service.Service at 0x2225d134c10>
chrome_portable_path = r"D:\GoogleChromePortable\GoogleChromePortable.exe"
option = Options()
option.binary_location = chrome_portable_path
# 啟動瀏覽器
driver = webdriver.Chrome()
# 加載 HTML 文件
driver.get(f"file://{path}") # 使用本地 HTML 文件的絕對路徑
# 使用不同的選取方式
# 1. By.TAG_NAME
element_by_tag_name = driver.find_element(By.TAG_NAME, "h1")
print("By.TAG_NAME:", element_by_tag_name.text)
# 2. By.ID
element_by_id = driver.find_element(By.ID, "username")
print("By.ID:", element_by_id.get_attribute("placeholder"))
# 3. By.CLASS_NAME
element_by_class_name = driver.find_element(By.CLASS_NAME, "submit-btn")
print("By.CLASS_NAME:", element_by_class_name.text)
# 4. By.NAME
element_by_name = driver.find_element(By.NAME, "password")
print("By.NAME:", element_by_name.get_attribute("placeholder"))
# 5. By.LINK_TEXT
element_by_link_text = driver.find_element(By.LINK_TEXT, "Visit Example")
print("By.LINK_TEXT:", element_by_link_text.get_attribute("href"))
# 關閉瀏覽器
driver.quit()
輸出結果:
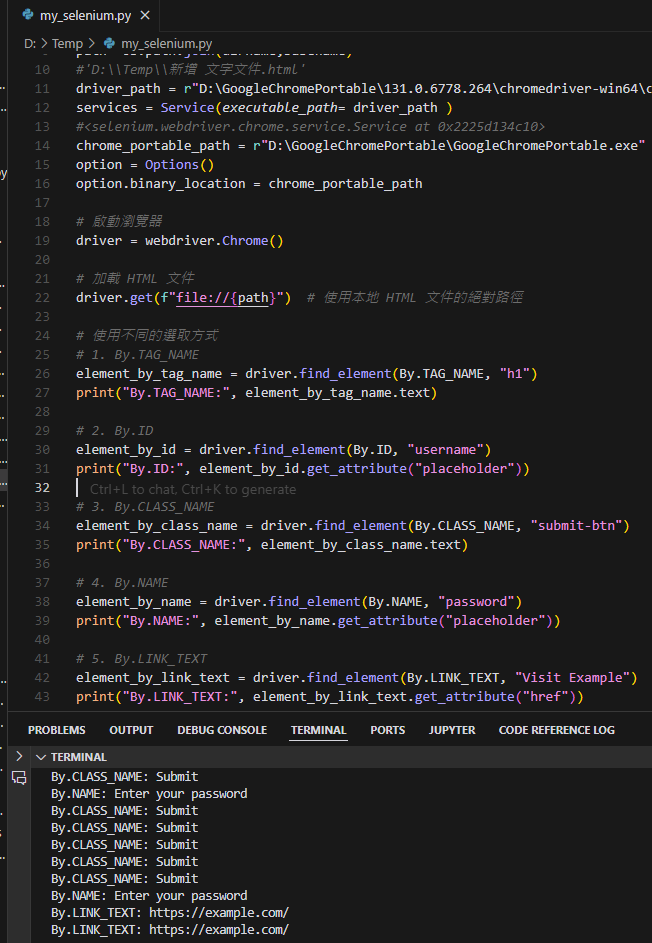
- 使用 CSS_SELECTOR 模擬其他選取方式
以下用 CSS_SELECTOR 模擬上述的選取方式。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.chrome.service import Service
import os
dirname = r"D:\Temp"
basename = "demo.html"
path= os.path.join(dirname,basename)
#'D:\\Temp\\新增 文字文件.html'
driver_path = r"D:\GoogleChromePortable\131.0.6778.264\chromedriver-win64\chromedriver.exe"
services = Service(executable_path= driver_path )
#<selenium.webdriver.chrome.service.Service at 0x2225d134c10>
chrome_portable_path = r"D:\GoogleChromePortable\GoogleChromePortable.exe"
option = Options()
option.binary_location = chrome_portable_path
# 啟動瀏覽器
driver = webdriver.Chrome()
# 加載 HTML 文件
driver.get(f"file://{path}") # 使用本地 HTML 文件的絕對路徑
# 使用 CSS_SELECTOR 模擬其他選取方式
# 1. 模擬 By.TAG_NAME
element_css_tag = driver.find_element(By.CSS_SELECTOR, "h1")
print("CSS_SELECTOR 模擬 By.TAG_NAME:", element_css_tag.text)
# 2. 模擬 By.ID
element_css_id = driver.find_element(By.CSS_SELECTOR, "#username")
print("CSS_SELECTOR 模擬 By.ID:", element_css_id.get_attribute("placeholder"))
# 3. 模擬 By.CLASS_NAME
element_css_class = driver.find_element(By.CSS_SELECTOR, ".submit-btn")
print("CSS_SELECTOR 模擬 By.CLASS_NAME:", element_css_class.text)
# 4. 模擬 By.NAME
element_css_name = driver.find_element(By.CSS_SELECTOR, "input[name='password']")
print("CSS_SELECTOR 模擬 By.NAME:", element_css_name.get_attribute("placeholder"))
# 5. 模擬 By.LINK_TEXT
# CSS_SELECTOR 無法直接模擬完整的 LINK_TEXT,但可以用屬性選擇器模擬
element_css_link = driver.find_element(By.CSS_SELECTOR, "a[href='https://example.com']")
print("CSS_SELECTOR 模擬 By.LINK_TEXT:", element_css_link.get_attribute("href"))
# 關閉瀏覽器
driver.quit()
輸出結果
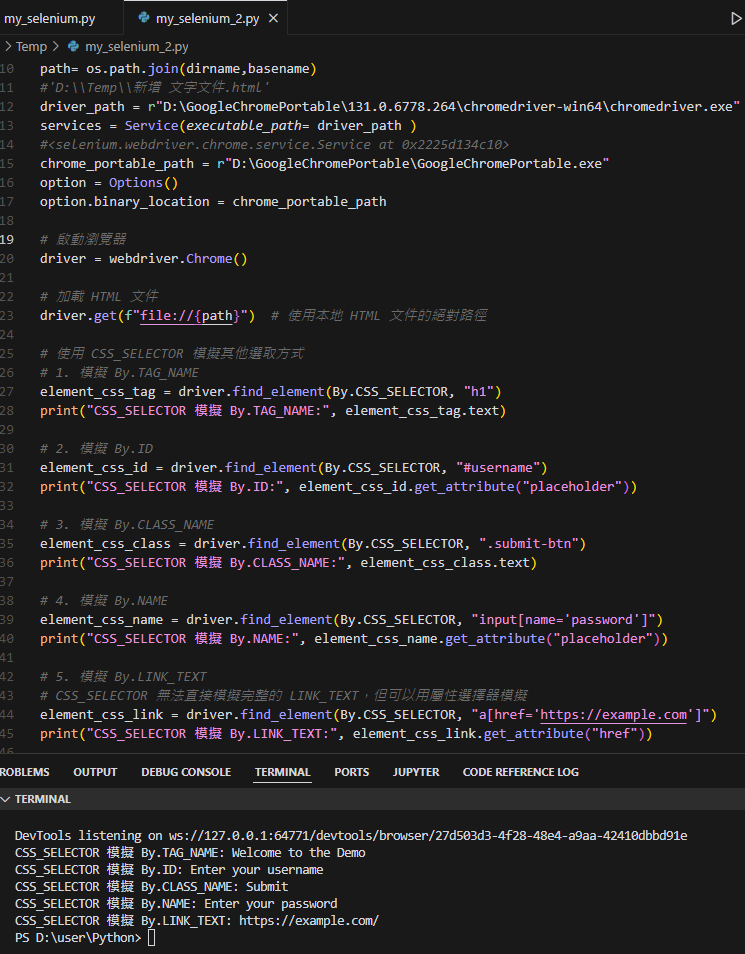
推薦hahow線上學習python: https://igrape.net/30afN
.find_element(By.TAG_NAME, “h1”)
.find_element(By.CSS_SELECTOR, “h1”)
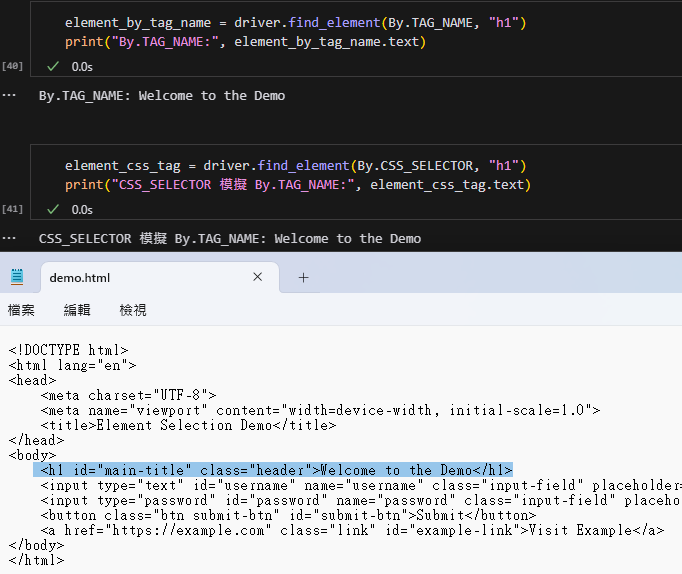
.find_element(By.ID, “username”)
.find_element(By.CSS_SELECTOR, “#username”)
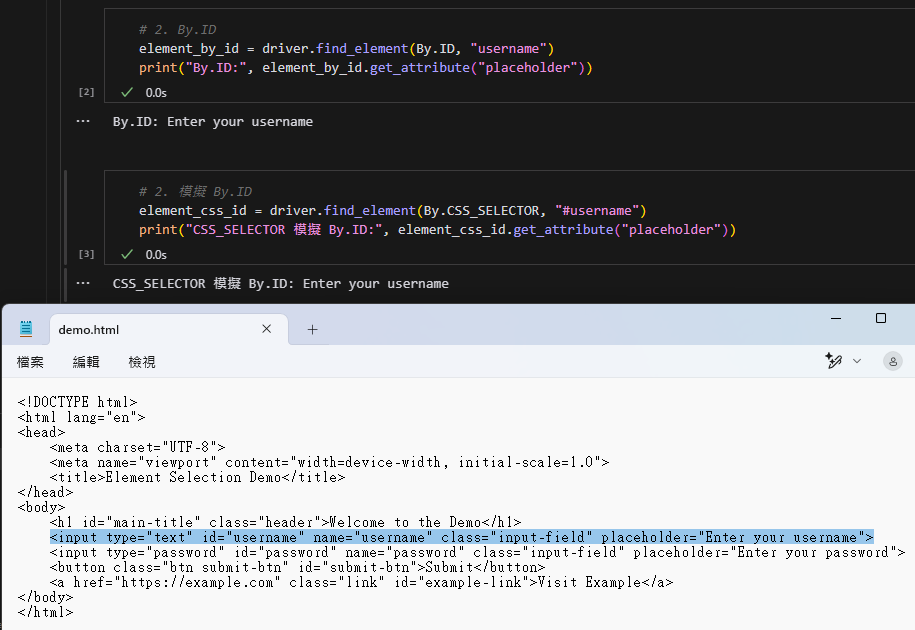
.find_element(By.CLASS_NAME, “submit-btn”)
.find_element(By.CSS_SELECTOR, “.submit-btn”)
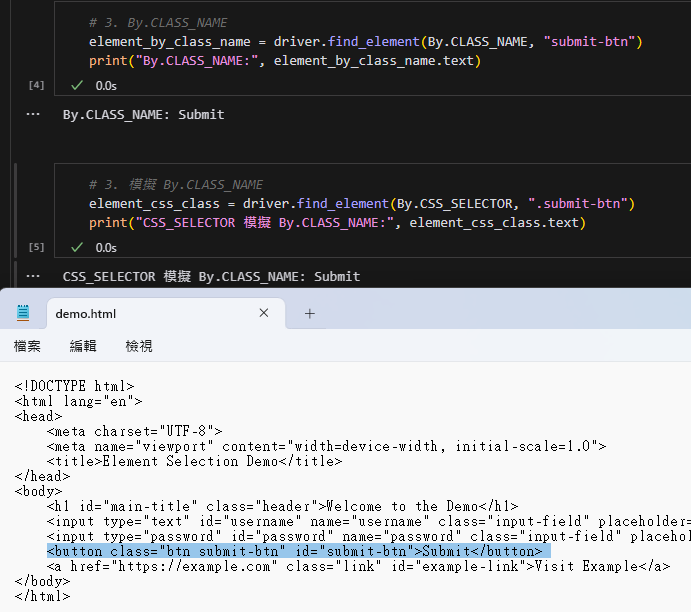
.find_element(By.NAME, “password”)
.find_element(By.CSS_SELECTOR, “input[name=’password’]”)
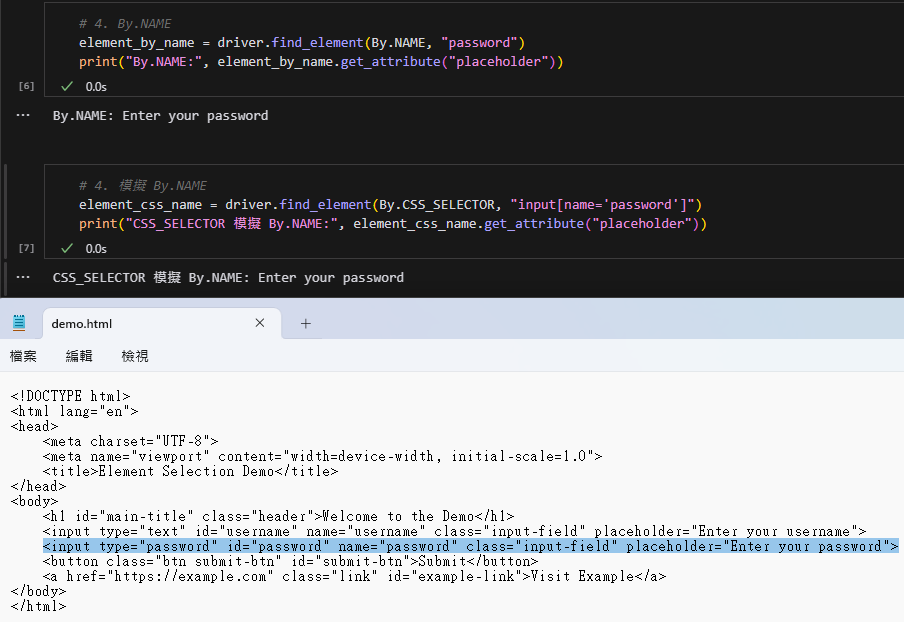
.find_element(By.LINK_TEXT, “Visit Example”)
.find_element(By.CSS_SELECTOR, “a[href=’https://example.com’]”)
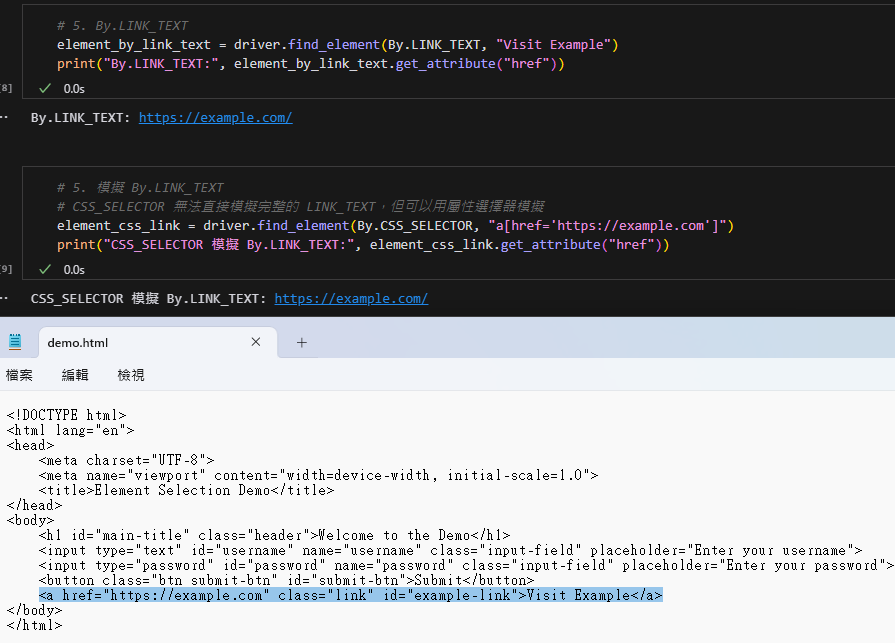
推薦hahow線上學習python: https://igrape.net/30afN
近期留言